I'm building a script that will ultimately take data from a csv file, populate a spreadsheet, use that spreadsheet to autofill a number of documents, and then automatically e-mail those documents to customers. It's also moving the documents from each time the script runs to a new folder with just that day's reports. I'm pretty new to Google Scripts, and this has been my learning project.
The steps I've got to work so far is the creation of documents for the spreadsheet with dummy data.
I ended up creating a second script to send e-mails which sends e-mails, but it is set up to look for all documents in the folder that are google docs, so it send a copy of every document to each customer. I thought that if I pointed the e-mail back to the original spreadsheet to grab just the correct document IDs (instead of the type) , I could only send customers the reports that belonged to them (all in PDF form). The step of creating a new folder and moving the documents into it afterwards works fine.
What I'm having an issue with is inputting data into my document ID column in the original spreadsheet. I have been able to watch it put the document ID of the first document into every row that has info to iterate over in the column, and then replace every row again with the second document's ID, etc.
I looked for ways to add data to a spreadsheet. Every method I've found so far involves creating a new column or row with new information from data within the spreadsheet. I need to put in data that I'm just now creating outside of the spreadsheet and then put it in the right place so I can point to it later.
I've gone over the methods within the documentation. It looks like .getCell.setvalue(variable) should work...if I could find a way to get the cell from the range (Which keeps showing me out of range).
function createDocument() {
var headers = Sheets.Spreadsheets.Values.get('17jXy9IlLt8C41tWEG5iQR31GjzOftlJs73y2L_0ZWNM', 'A1:P1');
var tactics = Sheets.Spreadsheets.Values.get('17jXy9IlLt8C41tWEG5iQR31GjzOftlJs73y2L_0ZWNM', 'A2:P');
var templateId = '1DU13OJHWyYnO5mLFovm97pWwXuU7ZTTDVJb2Mpdeebk';
for(var i = 0; i < tactics.values.length; i++){
var customer = tactics.values[i][0];
var pcname = tactics.values[i][1];
var date = tactics.values[i][2];
var virusvalue = tactics.values[i][3];
var cpuuse = tactics.values[i][4];
var ramuse = tactics.values[i][5];
var harddrive = tactics.values[i][6];
var netuse = tactics.values[i][7];
var downtime = tactics.values[i][8];
var cpuperform = tactics.values[i][9];
var ramperform = tactics.values[i][10];
var harddiskperform = tactics.values[i][11];
var reccomend = tactics.values[i][13];
var custID = tactics.values[i][14];
var newdoc = tactics.values[i][15];
//Make a copy of the template file
var documentID = DriveApp.getFileById(templateId).makeCopy(DriveApp.getFolderById('1zV-WpzUKoRurE9FnBcfjBygBA5rCO67I')).getId();
//rename the copied file
DriveApp.getFileById(documentID).setName('MCHA ' + customer + ' - ' + pcname);
Logger.log('value1 ' + documentID);
//THIS IS THE AREA I'M TRYING TO FIX
var ss = SpreadsheetApp.openById('113aqWVAjjUYCmI2oFc_BTbXkWMPFPjk_SschsKEx6qU');
var cell = ss.getRange('P2:P').getCell([i], [15]);
cell.setValue(documentID);
SpreadsheetApp.flush();
Logger.log('value2 ' + documentID);
//This area has code to replace the tags in the document with values from the spreadsheet. I cut it for not being relevant.
}
}
Obviously defining the range is just going to fill and autofill each cell. That code worked when I tried it
I originally tried using the variable from my earlier list for the autofill, but I've since realized that shouldn't work at all.
I tried to set the range of cells and then set the current cell by the same notation ([i][15] etc.) which throws an 'Range not found' error. I feel like I must be missing some syntax.
A link to the folder with all the documents is below, if that helps.
https://drive.google.com/drive/folders/1sRhti3R8R-Cym0hr2S4XkAVn3wyBbSRu?usp=sharing
I may not have entirely understood the problem you are facing. But I can see the cause of the 'Range not found' error.
Your loop starts with a value of 0 for i. This causes the script to look for a range called P0 in the first iteration.
One way to avoid that is to try :
var cell = ss.getRange("P"+(i+1));
For code efficiency, I'd also suggest moving some of the code outside the loop. For example, the following line runs each time in the loop. But it could be defined once outside the loop and then the variable ss could be reused inside the loop.
var ss = SpreadsheetApp.openById('113aqWVAjjUYCmI2oFc_BTbXkWMPFPjk_SschsKEx6qU');
Similarly, you could define the template file outside the loop and then sue it inside to make copies:
var templateFile = DriveApp.getFileById(templateId); // Outside the loop
And then inside the loop:
var documentID = templateFile.makeCopy(DriveApp.getFolderById('1zV-WpzUKoRurE9FnBcfjBygBA5rCO67I')).getId();
Google apps script best practices suggests minimising calls to the spreadsheet, i.e. get the data in one call, process it, and then post the data to the sheet in one call. More here.
Hope this helps.
I have a Google Sheet with 5 columns (First Name, Address, SKU, Quote, Status).
I have an apps script function (createQuote) which looks at the above variable's values from google sheet row and create a google document quote replacing the variables to values.
I use Zapier to insert row into my above google sheet.
What am struggling with-:
I need a way to trigger my createQuote function right when a new row is inserted via zapier (Google Sheet API call).
I tried playing with triggers but couldn't make it, any help is appreciated.
thank you
here is the code for my function-
function quoteCreator(){
docTemplate = "googledocidgoeshere"
docName = "Proposal"
var sheet = SpreadsheetApp.getActive().getSheetByName("Main")
var values = sheet.getDataRange().getValues()
var full_name = values[1][0]
var copyId = DriveApp.getFileById(docTemplate).makeCopy(docName+" for "+full_name).getId()
// Open the temporary document
var copyDoc = DocumentApp.openById(copyId);
// Get the document’s body section
var copyBody = copyDoc.getActiveSection();
// Replace place holder keys/tags,
copyBody.replaceText("keyFullName", full_name);
copyDoc.saveAndClose();
// Convert temporary document to PDF by using the getAs blob conversion
var pdf = DriveApp.getFileById(copyId).getAs("application/pdf");
// put the link of created quote in the quote column
var url = DocumentApp.openById(copyId).getUrl()
var last = sheet.getRange(2, 7, 1, 1).setValue(url)
}
Note-: I haven't put the loop yet in above, i'll do that once it starts working as per my requirements.
Changes made via Sheets API or Apps Script do not fire onEdit triggers. I give two workarounds for this.
Web app
Have whatever process updates the sheet also send a GET or POST request to your script, deployed as a web application. As an example, a GET version might access https://script.google.com/.../exec?run=quoteCreator
function doGet(e) {
if (e.parameter.run == "quoteCreator") {
quoteCreator();
return ContentService.createTextOutput("Quote updated");
}
else {
return ContentService.createTextOutput("Unrecognized command");
}
}
The web application should be published in a way that makes it possible for your other process to do the above; usually this means "everyone, even anonymous". If security is an issue, adding a token parameter may help, e.g., the URL would have &token=myToken where myToken is a string that the webapp will check using e.parameter.token.
GET method is used for illustration here, you may find that POST makes more sense for this operation.
Important: when execution is triggered by a GET or POST request, the methods getActive... are not available. You'll need to open any spreadsheets you need using their Id or URL (see openById, openByUrl).
Timed trigger
Have a function running on time intervals (say, every 5 minutes) that checks the number of rows in the sheet and fires quoteCreator if needed. The function checkNewRows stores the number of nonempty rows in Script Properties, so changes can be detected.
function checkNewRows() {
var sp = PropertiesService.getScriptProperties();
var oldRows = sp.getProperty("rows") || 0;
var newRows = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("Main").getLastRow();
if (newRows > oldRows) {
sp.setProperty("rows", newRows);
quoteCreator();
}
}
I'm doing multiple queries (find amount of open/closed issues, use the open/closed issues (per person) to create a leaderboard of developer issue closes, etc - find how many issues were closed this week and how many opened last week - what's the newest issue, what's the oldest issue) to GitHub's API and trying to get the resultant data written onto a Google Sheet. I'm using Google Apps Script Editor to do this.
I can get the JSON data from GitHub API to the console no problem, but I'm not sure how to then parse and write it to Google Sheets. How could I do this, please?
An example of a JSON data (this time it was "open issues" grabbed from http://github.com/vizorvr/patches/ )
[17-08-08 13:47:46:353 EEST] {"total_count":271,"incomplete_results":false,"items":[{"url":"https://api.github.com/repos/vizorvr/patches/issues/2035","repository_url":"https://api.github.com/repos/vizorvr/patches","labels_url":"https://api.github.com/repos/vizorvr/patches/issues/2035/labels{/name}","comments_url":"https://api.github.com/repos/vizorvr/patches/issues/2035/comments","events_url":"https://api.github.com/repos/vizorvr/patches/issues/2035/events","html_url":"https://github.com/vizorvr/patches/issues/2035","id":245953104,"number":2035,"title":"Figure out which Three.JS plugins are missing from Patches and add them","user":{"login":"esaruoho","id":4966687,"avatar_url":"https://avatars1.githubusercontent.com/u/4966687?v=4","gravatar_id":"","url":"https://api.github.com/users/esaruoho","html_url":"https://github.com/esaruoho","followers_url":"https://api.github.com/users/esaruoho/followers","following_url":"https://api.github.com/users/esaruoho/following{/other_user}","gists_url":"https://api.github.com/users/esaruoho/gists{/gist_id}","starred_url":"https://api.github.com/users/esaruoho/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/esaruoho/subscriptions","organizations_url":"https://api.github.com/users/esaruoho/orgs","repos_url":"https://api.github.com/users/esaruoho/repos","events_url":"https://api.github.com/users/esaruoho/events{/privacy}","received_events_url":"https://api.github.com/users/esaruoho/received_events","type":"User","site_admin":false},"labels":[],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2017-07-27T07:41:52Z","updated_at":"2017-07-27T07:41:52Z","closed_at":null,"body":"Go to http://threejs.org and compare the list of ThreeJS plugins in Patches and what is available on the ThreeJS site. Then implement them.","score":1.0},{"url":"https://api.github.com/repos/vizorvr/patches/issues/2034","repository_url":"https://api.github.com/repos/vizorvr/patches","labels_url":"https://api.github.com/repos/vizorvr/patches/issues/2034/labels{/name}","comments_url":"https://api.github.com/repos/vizorvr/patches/issues/2034/comments","events_url":"https://api.github.com/repos/vizorvr/patches/issues/2034/events","html_url":"https://github.com/vizorvr/patches/issues/2034","id":245952930,"number":2034,"title":"Inspect the Radial Point Geometry -plugin and figure out what it does or should do","user":{"login":"esaruoho","id":4966687,"avatar_url":"https://avatars1.githubusercontent.com/u/4966687?v=4","gravatar_id":"","url":"https://api.github.com/users/esaruoho","html_url":"https://github.com/esaruoho","followers_url":"https://api.github.com/users/esaruoho/followers","following_url":"https://api.github.com/users/esaruoho/following{/other_user}","gists_url":"https://api.github.com/users/esaruoho/gists{/gist_id}","starred_url":"https://api.github.com/users/esaruoho/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/esaruoho/subscriptions","organizations_url":"https://api.github.com/users/esaruoho/orgs","repos_url":"https://api.github.com/users/esaruoho/repos","events_url":"https://api.github.com/users/esaruoho/events{/privacy}","received_events_url":"https://api.github.com/users/esaruoho/received_events","type":"User","site_admin":false},"labels":[],"state":"open","locked":false,"assignee":null,"assignees":[],"milestone":null,"comments":0,"created_at":"2017-07-27T07:41:07Z","updated_at":"2017-07-27T07:41:07Z","closed_at":null,"body":"Go into Patches and add the Radial Point Geometry -patch.\r\n\r\nStudy what it does. Figure if it does anything. If it does something, come up with an example explaining how it should be used - then help with the popup documentation.\r\n\r\n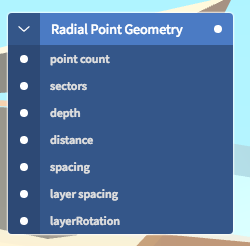\r\n","score":1.0},{"url":"https://api.github.com/repos/vizorvr/patches/issues/2023","repository_url":"https://api.github.com/repos/vizorvr/patches","labels_url":"https://api.github.com/repos/vizorvr/patches/issues/2023/labels{/name}","comments_url":"https://api.github.com/repos/vizorvr/patches/issues/2023/comments","events_url":"https://api.github.com/repos/vizorvr/patches/issues/2023/events","html_url":"https://github.com/vizorvr/patches/issues/2023","id":241289959,"number":2023,"title":"Shortcut: Shift-Tab: improve by opening \"Inspector->Properties\"","user":{"login":"esaruoho","id":4966687,"avatar_url":"https://avatars1.githubusercontent.com/u/4966687?v=4","gravatar_id":"","url":"https://api.github.com/users/esaruoho","html_url":"https://github.com/esaruoho","followers_url":"https://api.github.com/users/esaruoho/followers","following_url":"https://api.github.com/users/esaruoho/following{/other_user}","gists_url":"https://api.github.com/users/esaruoho/gists{/gist_id}","starred_url":"https://api.github.com/users/esaruoho/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/esaruoho/subscriptions","organizations_url":"https://api.github.com/users/esaruoho/orgs","repos_url":"https://api.github.com/users/esaruoho/repos","events_url":"https://api.github.com/users/esaruoho/events{/privacy}","received_events_url":"https://api.github.com/users/esaruoho/received_events","type":"User","site_admin":false},"labels":[{"id":165125694,"url":"https://api.github.com/repos/vizorvr/patches/labels/enhancement","name":"enhancement","color":"84b6eb","default":true},{"id":346495796,"url":"https://api.github.com/repos/vizorvr/patches/labels/user","name":"user","color":"fbca04","default":false},{"id":270777736,"url":"https://api.github.com/repos/vizorvr/patches/labels/UX","name":"UX","color":"5319e7","default":false}],"state":"open","locked":false,"assignee":{"login":"gmarinov","id":14101296,"avatar_url":"https://avatars0.githubusercontent.com/u/14101296?v=4","gravatar_id":"","url":"https://api.github.com/users/gmarinov","html_url":"https://github.com/gmarinov","followers_url":"https://api.github.com/users/gmarinov/followers","following_url":"https://api.github.com/users/gmarinov/following{/other_user}","gists_url":"https://api.github.com/users/gmarinov/gists{/gist_id}","starred_url":"https://api.github.com/users/gmarinov/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/gmarinov/subscriptions","organizations_url":"https://api.github.com/users/gmarinov/orgs","repos_url":"https://api.github.com/users/gmarinov/repos","events_url":"https://api.github.com/users/gmarinov/events{/privacy}","received_events_url":"https://api.github.com/users/gmarinov/received_events","type":"User","site_admin":false},"assignees":[{"login":"gmarinov","id":14101296,"avatar_url":"https://avatars0.githubusercontent.com/u/14101296?v=4","gravatar_id":"","url":"https://api.github.com/users/gmarinov","html_url":"https://github.com/gmarinov","followers_url":"https://api.github.com/users/gmarinov/followers","following_url":"https://api.github.com/users/gmarinov/following{/other_user}","gists_url":"https://api.github.com/users/gmarinov/gists{/gist_id}","starred_url":"https://api.github.com/users/gmarinov/starred{/owner}{/repo}","subscriptions_url":"https://api.github.com/users/gmarinov/subscriptions","organizations_url":"https://api.github.com/users/gmarinov/orgs","repos_url":"https://api.github.com/users/gmarinov/repos","events_url":"https://api.github.com/users/gmarinov/events{/privacy}","received_events_url":"https://api.github.com/users/gmarinov/received_events","type":"User","site_admin":false}],"milestone":null,"comments":0,"created_at":"2017-07-07T14:57:29Z","updated_at":"2017-07-07T15:44:19Z","closed_at":null,"body":"Improve Shift-Tab so that it opens the Inspector ( press I ) AND selects Properties-tab instead of Node.\r\n\r\n","score":1.0},{"url":"https://api.github.com/repos/vizorvr/patches/issues/2022","repository_url":"https://api.github.com/repos/vizorvr/patches","labels_url":"https://api.github.com/repos/vizorvr/patches/issues/2022/labels{/name}","comments_url":"https://api.github.com/repos/vizorvr/patches/issues/2022/comments","events_url":"https://api.github.com/repos/vizorvr/patches/issues/2022/events","html_url":"https://github.com/vizorvr/patches/issues/2022","id":24086639
I have, thus far, grabbed something called pullJSON to try and pull the data, which looks like this - however, unfortunately it seems destructive - it does not write to a specific sheet, just any old active sheet. I'm not sure how to modify it to write it to a specific sheet so that it'll work, so that's why I'm asking about how to parse stuff.
function pullJSON() {
var ss = SpreadsheetApp.getActiveSpreadsheet();
var sheets = ss.getSheets();
var sheet = ss.getActiveSheet();
var url="https://api.github.com/search/issues?q=repo:vizorvr/patches+state:closed"; // Paste your JSON URL here
var response = UrlFetchApp.fetch(url); // get feed
var dataAll = JSON.parse(response.getContentText()); //
var dataSet = dataAll;
var rows = [],
data;
for (i = 0; i < dataSet.length; i++) {
data = dataSet[i];
rows.push([data.id, data.name,data.email]); //your JSON entities here
}
dataRange = sheet.getRange(1, 1, rows.length, 3); // 3 Denotes total number of entites
dataRange.setValues(rows);
}
Edit 2
Was able to get the number of open issues in the repo, and the number of closed issues in the repo, but to dump them to a specific cell is still very vague for me.
var openPatches = "https://api.github.com/search/issues?q=repo:vizorvr/patches+state:open"
var closedPatches = "https://api.github.com/search/issues?q=repo:vizorvr/patches+state:closed"
var openResponse = UrlFetchApp.fetch(openPatches, {'muteHttpExceptions': true});
var Data = JSON.parse(openResponse.getContentText());
Logger.log("Open issues on Patches GitHub:")
Logger.log(Data.total_count)
var closedresponse = UrlFetchApp.fetch(closedPatches, {'muteHttpExceptions': true});
var data = JSON.parse(closedresponse.getContentText());
Logger.log("Closed issues on Patches GitHub:")
Logger.log(data.total_count)
Logger.log(Object.keys(data))
Could I in theory just write Data.total_count and data.total_count to different cells on a sheet, without having to have a google sheet open in another tab on the browser?
Based on your update, to write the data in to a specific sheet you need to use getSheetByName to write in to a specific sheet
var url ="<<SPREADSHEET URL>>";
var ss= SpreadsheetApp.openByUrl(url)
var sheet = ss.getSheetByName("<<SHEET NAME>>");
To store the values in different cell, you can define the range and set the values accordingly.
sheet.getRange("A1").setValue(Data.total_count); //Open issues
sheet.getRange("B1").setValue(data.total_count); //Closed issues
Apologies as I am a beginner to coding. I am interested in using Google Apps Script to automate the analysis of a Google Form response.
The simple example I have is for the spreadsheet of responses for a form asking people:
1) how many players there were?
2) where they finished [1st, 2nd, etc,]
On submission of the form I want to run a script that calculates how may points they received and inserts this value in the next available column (column E in this example).
I have tried writing my first Apps Script to automate this process, but without success.
SAMPLE CODE:
function onFormSubmit(e) {
var ss = SpreadsheetApp.getActiveSpreadsheet();
var sheet = ss.getSheetByName("Form Responses Master");
var players = e.values[2];
var place = e.values[3];
var positionPoints = e.values[4];
var positionPoints = (players - place + 1);
return positionPoints;
}
I know there are workarounds available by creating duplicate pages, but I was hoping someone might be able to advise me on how to code a solution in App Scripts, in the hope I might get a better understanding of the coding process.
You can write your appscript in the response collector spreadsheet itself instead of writing your code in form's script editor.
So, go to your response sheet and paste this code.
function myFunction()
{
var sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("Form Responses Master");
var rowNo = sheet.getLastRow();
var colNo = sheet.getLastColumn();
sheet.getRange(rowNo, colNo).setValue("=(C"+rowNo+"-D"+rowNo+")+1");
}
Now, go to Resources -> Current project triggers. Click on add new and set these values in drop downs: myFunction - From Spreadsheet - On form submit.
And you are done. Whenever a new response is submitted, position points will be calculated automatically.
Here,
variable sheet gets your active spreadsheet for different sheet operations which you can perform.
rowNo and colNo as seen in the code, simply fetches the value of last row/column respectively of spreadsheet in which something is written.
And, to set formula in column 'E', you can use setValue. Hence, "=(C"+rowNo+"-D"+rowNo+")+1" will be converted to (C2-D2)+1 in case of second row and so on for next rows.
getRange is simply used to tell the script that write formula inside particular cell.