i have this div class in style
.page-banner{
background-image:url(../images/background-sec1.png);
background-repeat:no-repeat;
and I've tried to create a new div in the same style with the same declarations but with a new name .page-banner-category.
.page-banner-category{
background-image:url(../images/background-sec1.png);
background-repeat:no-repeat;
and ...
<div class="page-banner-category">
but it does not work,what am i missing here?
Can you provide your HTML code as well to see how you use them? It is difficult to say right away what went wrong.
Also, in your CSS code you don't have to create 2 declarations, you can just use a multiple selector:
.page-banner, .page-banner-**category**{
background-image:url(../images/background-sec1.png);
background-repeat:no-repeat;
}
It will be easier down the road to update them when needed if behaviour is completely
here is.
<div class="page-banner-category col-md-10">
<?php echo single_cat_title('', false); ?>
<div class="theme-breadcrumb">
<?php niche_custom_breadcrumbs(); ?>
</div>
</div>
</div>
Was published before, this is for PHP, and what I try to do is just change the image banner in X category.
Thanks for you reply.
Your help would be immensely appreciated and thank you in advance to the audience.
I am having a small problem of CSS and .tpl files. I am currently building a site for a client using weebly cms. Which is great. However they are now using 'less' instead of normal css. This means they have partials and variables included now. Which I am unfamiliar with. I simply want to align some text to 'center' which is always a straight forward process in traditional CSS. In this case. the div class for the text in question lies within the .tpl file in 'partials' There is no class associated with the text in the normal CSS file.
I'd like to know if I could add the class within the normal CSS from the .tpl file. I have tried using the same class name from the .tpl within CSS using id tags and class tags i.e # and .
However with no success. Im sure it is relatively straight forward but I cannot get my little head around it.
Any help would be superb, Happy new year all!
Thank you
This is the .tpl code in question, as you can see the class names are simple.
wsite-com-product-price
wsite-com-price
wsite-come-catergory-product-price
<div class="wsite-com-product-price {{price_class}}">
<div class="wsite-com-price {{!
}}{{^is_featured}}wsite-com-category-product-price{{/is_featured}} {{!
}}{{#is_featured}}wsite-com-category-product-featured-price{{/is_featured}}">
{{{price_html}}}
</div>
The CSS relating is this: the first line is my attempt at making this work:
.wsite-com-price .wsite-com-product-price .wsite-com-category-product-price {
text-align: center;
}
I simply would just like to align the price to center:
The following is the HTML from view source on the page, (how i found the class names, but they are in .tpl file not the css!)
<div class="wsite-com-category-product-name wsite-com-link-text">
Merola Choker 1
</div>
</a>
<div class="wsite-com-product-price ">
<div class="wsite-com-price wsite-com-category-product-price ">
£125.00 - £165.00
</div>
<div class="wsite-com-sale-price wsite-com-category-product-price ">
£125.00 - £165.00
</div>
</div>
</div>
</div>
<div class="wsite-com-category-product wsite-com-column " data-id="5">
<div class="wsite-com-category-product-wrap ">
THANK YOU SO MUCH FOR ANYONE INTERESTED!
CORRECT ANSWERS SCREENSHOTS BELOW>>> THANKS!!!!
Try to use:
.wsite-com-category-product-price {text-align: center;}
As combinations of other classes might not always work.
Or:
.wsite-com-price, .wsite-com-product-price, .wsite-com-category-product-price {text-align: center}
To cover all price related classes.
.wsite-com-price .wsite-com-product-price .wsite-com-category-product-price {
text-align: center;
}
Here .wsite-com-price is expected to be parent class, which is not there in your tpl. (or did i missout).
.wsite-com-product-price, .wsite-com-category-product-price {
text-align: center;
}
Try this if, this works, they you missed the parent class.
If my HTML looks like this:
<div class="purely-aesthetic-wrapper">
<section>
…
</section>
</div>
…is it possible to omit the outside div from the HTML and do something like this (with LESS):
section {
enclose-with(.purely-aesthetic-wrapper);
}
Sorry if it's an obvious question but I'm not having much luck finding the answer.
Short answer is no, it's not possible.
LESS is css pre-processor and it has nothing to do with HTML/DOM-tree
Okay, bear with me because I'm new to WEB development.
I have an MVC 4.0 Web API application using Razor and Entity Framework 5 (C#).
One of my links takes me to a page which displays data from the EF.
I added an HTML button to this page called "Export to Excel."
I want to center this button on the screen, above the report results.
What is the correct approach for this and how do I do it? I need to understand the PROPER architecture for files and code.
Do I use CSS? If so, where do I store the file in the solution? How do I use it on my page?
Do I do something specific to Razor?
Should I simply use HTML tags like
Again, all I want to do is center a button on the web page.
Can someone help me with a step by step process to do this correctly?
I'm just having a hard time figuring out where to put code and files (basically how to structure the application properly).
You can use CSS.
.centerAlign {
text-align: center;
}
In your view you can then apply the centerAlign class to the button:
<button class='centerAlign' />
You can also make a custom HTML Helper that will automatically apply the class for you.
namespace YourApplication.Helpers
{
public static class ButtonExtensions
{
public static string ButtonCenter(this HtmlHelper helper, string value)
{
return String.Format("<button class='centerAlign'> {0} </button>", value);
}
}
}
Then in your view you could do:
#Html.ButtonCenter("Click me");
I know there is an answer, but this is a really common way to center objects margin: 0 auto. Since the answer by itself doesn't really explain, I'll provide some detail.
When you build your View it is marked as a .cshtml file. Your View will contain a series of HTML or Hyper Text Markup Language. What you are doing is utilizing a particular Element called a div. These are used to help build a structure or layout for your site.
<div id="Origin">
<div class="origin-container">
<div class="header-style">
<div id="Origin-Header">
<div class="header-container">
// Inside Header Elements
</div>
</div>
</div>
</div>
</div>
So essentially this is a Site Structure. You'll notice that I have a tendency to inner-wrap a lot of my Elements. That is because it makes it easier to customize and style my layout, making it more customizable.
If your thinking "How is it more customizable?" Your partially correct, this HTML is simply a structure- The customization will come from your Stylesheet, Cascading Stylesheet to be exact.
Your HTML will call this Stylesheet to help adapt your layout to give a consistent appearance. So if you'd like to center your header you would put in your stylesheet:
#Origin-Header {
margin: 0 auto;
}
What the command is stating is three things:
Margin: These are the page margins.
0: Is the pixel difference for Top and Bottom.
Auto: The left and right pixels.
So rather then a top, bottom, left, and right they are all merged together in the short-hand. You'll have a lot of additional control to your layout as well through your stylesheet. Bare in mind that this is manipulating those div tags. If your trying to align a particular object, it would work identical but rather then focus on the element- You would point it to your html object.
But I hope that helps.
I do not know if it is good practice, but the only way I have been able to center entire controls like a button in HTML was to do something like
.btn{
margin-left:auto;
margin-right:auto;
}
I have been able to center the control by setting the button's class to "btn" and using the above css. The text-align property has never really worked for me in HTML.
Try this:
[i guess is this what you are looking for]
check LIVE DEMO on jsfiddle
HTML
<section class="iHaveBorder">
<h3>Help me here!...Center stuff...</h3>
<div class="myWorderfullDiv iAmRed">
your report here...
</div>
<center>
<button>DoIt</button>
</center>
</section>
<h1>::::::OR:::::</h1>
<section class="iHaveBorder">
<h3>Help me here!...Center stuff...</h3>
<div class="myWorderfullDiv iAmBlue">your report here...</div>
<div style="text-align: center;" >
<button>DoIt</button>
</div>
</section>
<h1>::::::OR relative:::::</h1>
<section class="iHaveBorder iFeetIn">
<h3>Help me here!...Center stuff...</h3>
<div class="myWorderfullDiv iAmGreen">your report here...</div>
<div class="iWant2BeCenter">
<button>DoIt</button>
</div>
</section>
CSS:
.myWorderfullDiv{
height: 80px; width: 200px;
}
.iAmBlue{background-color: blue}
.iAmRed{background-color: red}
.iAmGreen{background-color: green}
.iHaveBorder{border:2px black solid;}
.iFeetIn{display: inline-block;}
.iWant2BeCenter{text-align: center;}
check LIVE DEMO on jsfiddle
I am making a site that publishes articles in issues each month. It is straightforward, and I think using a Markdown editor (like the WMD one here in Stack Overflow) would be perfect.
However, they do need the ability to have images right-aligned in a given paragraph.
I can't see a way to do that with the current system - is it possible?
You can embed HTML in Markdown, so you can do something like this:
<img style="float: right;" src="whatever.jpg">
Continue markdown text...
I found a nice solution in pure Markdown with a little CSS 3 hack :-)



Follow the CSS 3 code float image on the left or right, when the image alt ends with < or >.
img[alt$=">"] {
float: right;
}
img[alt$="<"] {
float: left;
}
img[alt$="><"] {
display: block;
max-width: 100%;
height: auto;
margin: auto;
float: none!important;
}
Many Markdown "extra" processors support attributes. So you can include a class name like so (PHP Markdown Extra):
{.callout}
or, alternatively (Maruku, Kramdown, Python Markdown):
{: .callout}
Then, of course, you can use a stylesheet the proper way:
.callout {
float: right;
}
If yours supports this syntax, it gives you the best of both worlds: no embedded markup, and a stylesheet abstract enough to not need to be modified by your content editor.
I have an alternative to the methods above that used the ALT tag and a CSS selector on the alt tag... Instead, add a URL hash like this:
First your Markdown image code:



Note the added URL hash #center.
Now add this rule in CSS using CSS 3 attribute selectors to select images with a certain path.
img[src*='#left'] {
float: left;
}
img[src*='#right'] {
float: right;
}
img[src*='#center'] {
display: block;
margin: auto;
}
You should be able to use a URL hash like this almost like defining a class name and it isn't a misuse of the ALT tag like some people had commented about for that solution. It also won't require any additional extensions. Do one for float right and left as well or any other styles you might want.
I like to be super lazy by using tables to align images with the vertical pipe (|) syntax. This is supported by some Markdown flavours (and is also supported by Textile if that floats your boat):
| I am text to the left |  |
or
|  | I am text to the right |
It is not the most flexible solution, but it is good for most of my simple needs, is easy to read in markdown format, and you don't need to remember any CSS or raw HTML.
Embedding CSS is bad:

CSS in another file:
img[alt=Flowers] { float: right; }
<div style="float:left;margin:0 10px 10px 0" markdown="1">

</div>
The attribute markdown possibility inside Markdown.
Even cleaner would be to just put p#given img { float: right } in the style sheet, or in the <head> and wrapped in style tags. Then, just use the markdown .
I liked learnvst's answer of using the tables because it is quite readable (which is one purpose of writing Markdown).
However, in the case of GitBook's Markdown parser I had to, in addition to an empty header line, add a separator line under it, for the table to be recognized and properly rendered:
| - | - |
|---|---|
| I am text to the left |  |
|  | I am text to the right |
Separator lines need to include at least three dashes --- .
If you implement it in Python, there is an extension that lets you add HTML key/value pairs, and class/id labels. The syntax is for this is:
{: style="float:right"}
Or, if embedded styling doesn't float your boat,
{: .floatright}
with a corresponding stylesheet, stylish.css:
.floatright {
float: right;
/* etc. */
}
As greg said you can embed HTML content in Markdown, but one of the points of Markdown is to avoid having to have extensive (or any, for that matter) CSS/HTML markup knowledge, right? This is what I do:
In my Markdown file I simply instruct all my wiki editors to embed wrap all images with something that looks like this:
'<div> // Put image here </div>`
(of course.. they don't know what <div> means, but that shouldn't matter)
So the Markdown file looks like this:
<div>
![optional image description][1]
</div>
[1]: /image/path
And in the CSS content that wraps the whole page I can do whatever I want with the image tag:
img {
float: right;
}
Of course you can do more with the CSS content... (in this particular case, wrapping the img tag with div prevents other text from wrapping against the image... this is just an example, though), but IMHO the point of Markdown is that you don't want potentially non-technical people getting into the ins and outs of CSS/HTML.. it's up to you as a web developer to make your CSS content that wraps the page as generic and clean as possible, but then again your editors need not know about that.
I had the same task, and I aligned my images to the right by adding this:
<div style="text-align: right"><img src="/default/image/sms.png" width="100" /></div>
For aligning your image to the left or center, replace
<div style="text-align: right">
with
<div style="text-align: center">
<div style="text-align: left">
You can directly use align property:
<img align="right" width="100" height="100" src="https://images.unsplash.com/photo-1650620109005-099c2de720f8?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxlZGl0b3JpYWwtZmVlZHwxM3x8fGVufDB8fHx8&auto=format&fit=crop&w=500&q=60">
The best and most customizable option:
<div style="display:flex; align-items: center;">
<div style="flex:1">
<img src="https://www.researchgate.net/profile/Jinsong-Chong/publication/233165295/figure/fig5/AS:667635838640135#1536188196882/Initial-contour-Figure-9-Detection-result-in-low-resolution-image-in-low-resolution-image.ppm"/>
</div>
<div style="flex:1;padding-left:10px;">
<img src="https://www.researchgate.net/profile/Miguel-Vega-4/publication/228966464/figure/fig1/AS:669376512544781#1536603205341/a-Observed-low-resolution-multispectral-image-b-Panchromatic-image-c.ppm" />
</div>
</div>
This will align the first to the left, and the second to the right. Works for more than 2 images too.
For a simple approach to just indenting your image a bit, just use some non-breaking spaces with an img element. E.g., <img src="https://user-images.githubusercontent.com/123456/123456789-3aabedfe-deab-4242-97a0-a6641e675e68.png" />
Align image and text side-by-side as part of a paragraph in a single block, within a warning box.
<div class="warning" style='background-color:#EDF2F7; color:#1A2067; border-left: solid #718096 4px; border-radius: 4px;'>
<p style='padding:0.7em; margin-left:0.7em; display: inline-block;'>
<img src="typora_images/image-20211028083121348.png" style="zoom:70%; float:right; padding:0.7em"/>
<b>isomorphism</b> → In mathematics, an isomorphism is a structure-preserving mapping between two structures of the same type that can be reversed by an inverse mapping.<br>
</p>
</div>
Output :
I think the easiest solution is to directly specify align="right":
<img align="right" src=/logo.png" alt="logo" width="100"/>
this work for me
<p align="center">
<img src="/LogoOfficial.png" width="300" >
</p>
Simplest is to wrap the image in a center tag, like so ...
<center>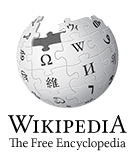</center>
Anything to do with Markdown can be tested here - http://daringfireball.net/projects/markdown/dingus
Sure, <center> may be deprecated, but it's simple and it works!